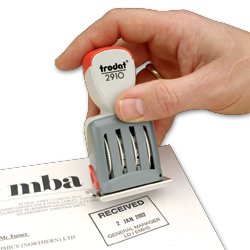
During development, generally your testers will be running a version of the game that could be a number of days old.
By the time you’ve got back bug reports there is likely to have been significant progress on the game since. There’s every chance during interim development that bugs could have been fixed before QA found them. Also, the goalposts could be moved; some aspect of the title may have been significantly changed and that bug report you got no longer makes any sense.
For these reasons it’s imperative that when you get back bugs, you know what version of the game they were running. There’s a number of ways you can address this:
Version Numbering
- Can be in various forms. Could possibly be a hard coded string, some piece of built data, or the version number built into a PC executable header.
- Many PC applications shipped just use simple version numbering to denote their builds. You still see a lot of “beta version 2.0.1.1123” style versioning on commercial software sitting out on webpages.
- Manually updating the minor revisions each time is an easy thing to forget. It is possible to set up most development environments to auto-increment this number. If there’s no native support, it can usually be hacked in as a pre-build step.
Source Control Revision
- There’s dozens of different pieces of source control software. With the majority of them it’s possible to synchronize to an explicit revision of the source tree. The revision number a game was built from can be used to identify a build.
- As a pre-build step it is possible to automatically embed this revision number into the game.
- Some source control software doesn’t have any sort of global revision numbering. Visual Sourcesafe springs to mind; that one only logs revision histories on a per-file basis. A single revision number to identify a particular build of the game is not possible. For Sourcesafe though it is possible to apply a label to the whole source tree. That could be done with each significant test build, with a version number of some kind.
Date/Time Stamping
- Simply the date and time at which the build was created. Similarly to the source control methods, you can easily pinpoint exactly what code and data made it into the particular build of the game.
- It falls down a little if your source control still accepts submissions, and you have other users submitting code whilst a build is being created. When cross referencing the date and time you actually built the game, it could appear that some changes got in that actually didn’t. This is no big deal if you lock out your source control whilst making a build for testing, or if you only have one person on the project.
- Don’t use the timestamp of the executable itself! Aside from easily being able to be overwritten (the create and modified dates could be blitzed over for example, with particular unzipping or CD burning software). On the Xbox with XNA it’s not actually possible to inspect the executable’s date using the System.IO calls.
- It is generally possible in most development environments to embed the current date and time into code. In C# this is actually possible in a very nice, clean manner. Updated intelligently each time you build an executable.
With your average XNA game you’ve got no QA department to rely on. Most testing would probably be done by the developers, and their friends and family. The easier and more transparent this identification-marking is, the better.
Automatic versioning in C#
C# does provide automatic versioning in the form of an implicit change to the assembly version. For a newly created XNA project you’ll have an ‘Assembly.cs’ file. At the bottom is the version number:
// Version information for an assembly consists of the following four values:
//
// Major Version
// Minor Version
// Build Number
// Revision
//
[assembly: AssemblyVersion("1.0.0.0")]
If you change the line to the following instead:
[assembly: AssemblyVersion("1.0.*")]
The last two numbers in the version will now be automatically filled in when the assembly is compiled. The numbers it fills in with are based on the current system date and time. So by doing this you’re effectively able to timestamp the built date of your game into the version number of the assembly. Both PC and Xbox are able to grab this version number, so it can be displayed within the game or on any sort of ‘game has crashed’ screen you have.
The first two numbers, the major and minor version can still be used for their usual purpose. If you’re so inclined.
The ‘build number’ and ‘revision’ portions of the version can be grabbed and converted to a C# DateTime class, with the following code:
private DateTime DateCompiled()
{
// The assembly version must have the last two numbers removed and replaced with *
// It should look something like this:
// [assembly: AssemblyVersion("1.0.*")]
//Build dates start from 01/01/2000
System.DateTime result = DateTime.Parse("1/1/2000");
//Retrieve the version information from the assembly from which this code is being executed
System.Version version = System.Reflection.Assembly.GetExecutingAssembly().GetName().Version;
//Add the number of days (build)
result = result.AddDays(version.Build);
//Add the number of seconds since midnight (revision) multiplied by 2
result = result.AddSeconds(version.Revision * 2);
//If we're currently in daylight saving time add an extra hour
if (TimeZone.IsDaylightSavingTime(System.DateTime.Now,
TimeZone.CurrentTimeZone.GetDaylightChanges(System.DateTime.Now.Year)))
{
result = result.AddHours(1);
}
return result;
}
Courtesy of this post from dotnetfreak.co.uk of equivalent VB.NET code.
So, this is what I’m going with to tag my game builds. It covers me for what I need, and requires zero maintenance.
Note:
It is mentioned on a number of webpages that the ‘build number’ and ‘revision’ numbers don’t update on each build. It’s said that they only update each time you actually start the Visual Studio application.
However, I haven’t found this to be the case with Visual Studio 2008. Both the PC and Xbox XNA projects update this just fine. I can edit any .cs file in my project, and the game gets a new timestamp. I believe that the posts about the bad behavior were with Visual Studio 2005 and 2003.
XNA content pipeline files though, do not affect the timestamp at all. This makes total sense though, as the binary xnb data files aren’t part of the actual executable assembly.
References:
- http://dotnetfreak.co.uk/blog/archive/2004/07/08/determining-the-build-date-of-an-assembly.aspx
- http://stackoverflow.com/questions/324245/aspnet-show-application-build-dateinfo-at-the-bottom-of-the-screen
- http://msdn.microsoft.com/en-us/library/system.reflection.assemblyversionattribute.assemblyversionattribute.aspx
Comments
How you find ideas for articles, I am always lack of new ideas for articles. Some tips would be great
[…] something is changed, mostly at the time of customer acceptance tests. I found following code from here. […]